[React] image 확대/축소(라이브러리 사용)
2023. 2. 15. 20:17
프로그래밍/React
image를 마우스 wheel로 확대/축소하는 기능 구현 react-zoom-pan-pinch 라이브러리 간단한 사용법 설치 npm i react-zoom-pan-pinch 사용법 import { TransformWrapper, TransformComponent } from "react-zoom-pan-pinch"; const ZoomInOut = () => { return ( ); }; export default ZoomInOut; 참고 자료 : https://www.npmjs.com/package/react-zoom-pan-pinch
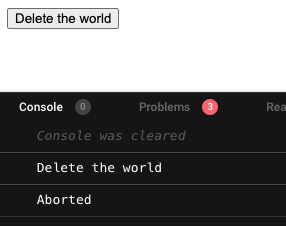
[React] React Hooks (11) - useConfirm, usePreventLeave
2023. 2. 8. 20:34
프로그래밍/React
useConfirm, usePreventLeave useConfirm, usePreventLeave를 간단하게 hooks로 만들어보자 useConfirm 예시 1 버튼 클릭 시 Are you sure 라는 confirm 창이 나오고 확인을 누르면 Delete the world 출력 취소를 누르면 Aborted 출력되는 간단한 함수형 프로그래밍 const useConfirm = (message = "", onConfirm, onCancel) => { if (!onConfirm && typeof onConfirm !== "function") { return; } if (onCancel && typeof onCancel !== "function") { return; } const confirmAction = ..

[React] React Hooks (10) - useNotification
2023. 2. 7. 20:07
프로그래밍/React
useNotification 브라우저 알람 이벤트를 hooks로 만들어보자 useNotification 예시 Hello 버튼 클릭시 브라우저 알람뜸 이때 알람이 허용된 경우에만 알람 확인 가능 const useNotification = (title, options) => { if (!("Notification" in window)) { return; } const fireNotif = () => { if (Notification.permission !== "granted") { Notification.requestPermission().then((permission) => { if (permission === "granted") { new Notification(title, options); } else..
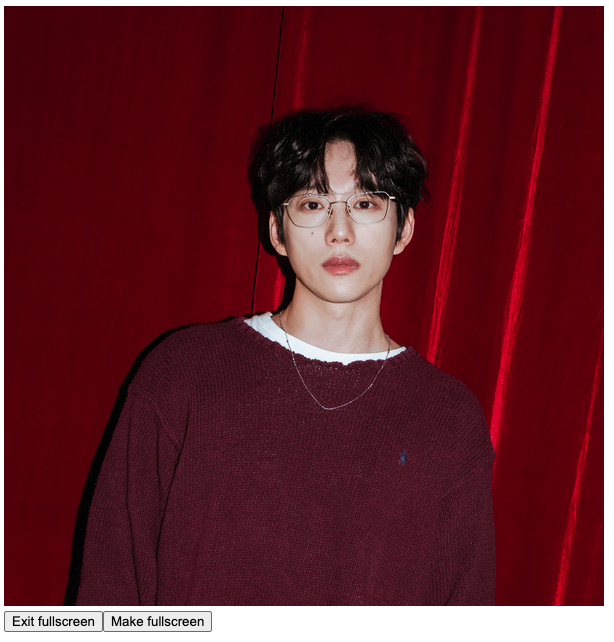
[React] React Hooks (9) - useFullscreen
2023. 2. 6. 20:47
프로그래밍/React
useFullscreen 이미지를 Fullscreen으로 만드는 hooks를 만들어보자 useFullscreen Make fullscreen 버튼 클릭시 이미지 fullscreen, Exit fullscreen 버튼 클릭시 fullscreen 빠져나오기 const useFullscreen = () => { const element = useRef(); const triggerFull = () => { if (element.current) { element.current.requestFullscreen(); } }; const exitFull = () => { document.exitFullscreen(); }; return { element, triggerFull, exitFull }; }; const ..
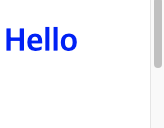
[React] React Hooks (8) - useScroll
2023. 2. 3. 20:26
프로그래밍/React
useScroll hooks를 사용해서 스크롤을 했을때 이벤트를 발생시키기 useScroll 예시 스크롤을 하면 color가 blue에서 red로 바뀌는 이벤트 const useScroll = () => { const [state, setState] = useState({ x: 0, y: 0 }); const onScroll = () => { setState({ y: window.scrollY, x: window.scrollX }); }; useEffect(() => { window.addEventListener("scroll", onScroll); return () => window.removeEventListener("scroll", onScroll); }, []); return state; }; c..
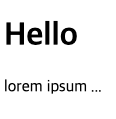
[React] React Hooks (7) - useFadeIn
2023. 2. 2. 20:11
프로그래밍/React
useFadeIn 서서히 나타나는 이벤트를 hooks로 만들어보기 useFadeIn 예시 3초 뒤 Hello, 15초 뒤 lorem ipsum...이 화면에 뜸 const useFadeIn = (duration = 1, delay = 0) => { if (typeof duration !== "number" || typeof delay !== "number") { return; } const element = useRef(); useEffect(() => { if (element.current) { const { current } = element; current.style.transition = `opacity ${duration}s ease-in-out ${delay}s`; current.style.o..
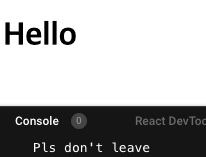
[React] React Hooks (6) - useBeforeLeave
2023. 2. 1. 20:48
프로그래밍/React
useBeforeLeave 탭을 닫을 때 실행되는 function 생성하기 구체적으로는 마우스의 위치를 확인해서 마우스가 탭쪽으로 갔을때 실행되는 함수임 useBeforeLeave 예시 마우스를 상단 탭 위로 가져가면 사진의 콘솔 메시지가 뜸 const useBeforeLeave = (onBefore) => { if (typeof onBefore !== "function") { return; } const handle = (event) => { const { clientY } = event; if (clientY { document.addEventListener("mouseleave", handle); return () => document.removeEventListener("mouseleave", h..
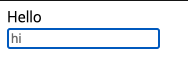
[React] React Hooks (5) - useRef
2023. 1. 31. 20:09
프로그래밍/React
useRef reference는 기본적으로 component의 어떤 부분을 선택할 수 있는 방법 document.getElementByID()와 같은 역할 useRef 예시1 const App = () => { const input = useRef(); setTimeout(() => input.current.focus(), 5000); return ( Hello ); }; 5초 뒤 input이 선택되어 focus 됨 useRef 예시2 const useClick = (onClick) => { const element = useRef(); useEffect(() => { if(element.current){ element.current.addEventListener("click", onClick); } r..