[Javascript] 배열을 문자열로 합치기
2023. 8. 24. 20:18
프로그래밍/JavaScript
배열을 문자열로 합치는 방법 1. join() 배열을 하나의 문자열로 리턴 받을 때 구분값을 줄 수 있음 const arr = new Array('하나', '둘', '셋'); console.log(arr.join());// 하나,둘,셋 console.log(arr.join(''));// 하나둘셋 console.log(arr.join(' '));// 하나 둘 셋 console.log(arr.join(', '));// 하나, 둘, 셋 console.log(arr.join('-'));// 하나-둘-셋 2. toString() 콤마(,)로 구분된 하나의 문자열 리턴 const arr = new Array('하나', '둘', '셋'); console.log(arr.toString());// 하나,둘,셋
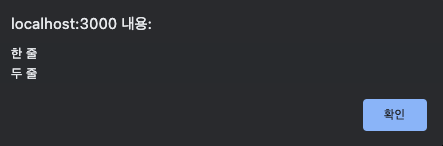
[Javascript] alert 창 줄바꿈
2023. 8. 4. 20:28
프로그래밍/JavaScript
alert 창 줄바꿈 줄바꿈을 위해서 \n으로 개행을 해주면 됨 예시 alert('한 줄\n두 줄');
[Javascript] 자바스크립트 slice와 splice 비교
2023. 7. 7. 20:01
프로그래밍/JavaScript
slice 배열의 일부분을 추출하여 새로운 배열을 반환 원본 배열은 변경되지 않음 사용 방법 array.slice(startIndex, endIndex); startIndex - 추출 시작 인덱스 (포함). 음수 값이면 배열의 끝에서부터 카운트 endIndex - 추출 종료 인덱스 (제외). 생략하면 배열의 끝까지 추출. 음수 값이면 배열의 끝에서부터 카운트 예시 const numbers = [1, 2, 3, 4, 5]; const slicedNumbers = numbers.slice(1, 4); console.log(slicedNumbers); // [2, 3, 4] splice 배열에서 요소를 추가, 삭제 또는 교체하는데 사용 원본 배열을 수정함 사용 방법 array.splice(startIndex,..
[Javascript] 숫자인지 체크하는 방법
2023. 7. 6. 20:30
프로그래밍/JavaScript
isNaN() isNaN() 함수는 주어진 값이 "Not-a-Number"인지 여부를 판별하는 함수 전달된 값이 숫자가 아닌 경우 true를 반환, 숫자인 경우 false를 반환 예시 console.log(isNaN(123)); // 출력: false console.log(isNaN('Hello')); // 출력: true console.log(isNaN('123')); // 출력: false console.log(isNaN('123abc')); // 출력: true console.log(isNaN(NaN)); // 출력: true console.log(isNaN(null)); // 출력: false console.log(isNaN(undefined)); // 출력: true isNaN(123): 숫자 ..
[Javascript] url 파일 다운로드
2023. 6. 28. 20:18
프로그래밍/JavaScript
url 파일 다운로드 javascript 혹은 react에서 파일 다운로드 하는 방법 예시 const handleDownload = async () => { // URL에서 GET 요청 보내기 fetch('다운로드 할 file url', { method: 'GET', }) // 응답 데이터를 블롭(Blob) 객체로 변환 .then((response) => response.blob()) .then((blob) => { // 블롭(Blob) 객체 생성하고 URL을 생성 const url = window.URL.createObjectURL(blob); const link = document.createElement('a'); // 생성한 URL과 다운로드할 파일명 설정 link.setAttribute('hre..
[Javascript] export 'default' (imported as 'XLSX') was not found in 'xlsx' 해결 방법
2023. 6. 26. 20:21
프로그래밍/JavaScript
export 'default' (imported as 'XLSX') was not found in 'xlsx' 에러 원인 import XLSX from 'xlsx'; // 중략... const handleFileUpload = (event) => { const file = event.target.files[0]; const reader = new FileReader(); reader.onload = (e) => { const data = new Uint8Array(e.target.result); const workbook = XLSX.read(data, { type: 'array' }); // 첫 번째 시트를 가져옴 const worksheet = workbook.Sheets[workbook.SheetN..
[Javascript] 자바스크립트, 리액트 xlsx 라이브러리 사용법
2023. 6. 23. 20:11
프로그래밍/JavaScript
xlsx 라이브러리 사용법 간단 정리 리액트에서 엑셀 업로드해서 데이터 가져오기위해 사용했던 라이브러리 사용법 설치하기 npm install xlsx # or yarn add xlsx 사용하기 업로드 하는 코드만 정리함 import React from 'react'; import * as XLSX from 'xlsx'; const ExcelUploader = () => { const handleFileUpload = (event) => { const file = event.target.files[0]; const reader = new FileReader(); reader.onload = (e) => { const data = new Uint8Array(e.target.result); const work..
[JavaScript] undefined, null 체크
2023. 5. 24. 20:57
프로그래밍/JavaScript
undefined 체크 if (typeof str === 'undefined') {...} null 체크 if (str === null) {...} 값 존재 여부 체크 if (typeof str === 'undefined' || str === null || str === '') { // 값이 없는 경우 실행될 코드 }