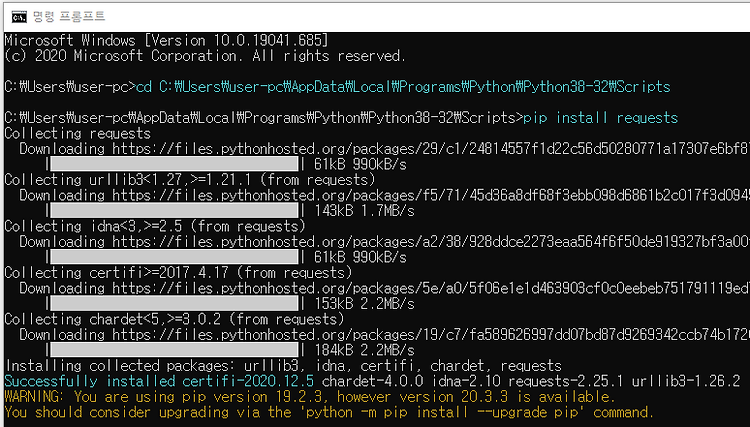
web Scrapping을 위한 requests와 BeautifulSoup 설치
2020. 12. 23. 12:07
프로그래밍/Python
웹에서 정보 가져오기 requests 모듈 파이썬 requests 모듈은 간편한 HTTP 요청처리를 위해 사용하는 모듈 requests 모듈 설치 requests 모듈을 사용하기 위해서는 pip를 통해 설치를 해야함 pip는 python 3.4이상 버전부터는 자동으로 같이 설치가 되기 때문에 따로 설치할 필요없음 1️⃣ cmd(명령 프롬프트) 실행 2️⃣ pip로 설치하기 위해 파이썬 하위에 있는 Scripts로 이동 이동할 때는 cd(Change Directory) 명령어 입력 👉 cd C:\Users\user-pc\AppData\Local\Programs\Python\Python38-32\Scripts 3️⃣ pip install requests 라고 입력하여 설치 진행 👉 pip install re..
개발환경 세팅
2020. 12. 23. 11:25
프로그래밍/Python
Python 세팅 1️⃣ 구글에서 python 검색 https://www.python.org/ Welcome to Python.org The official home of the Python Programming Language www.python.org 2️⃣ Download 메뉴에서 3.xx 버전 설치하기 Add Python 3.8 to PATH 꼭 체크하기
Modules
2020. 12. 22. 18:50
프로그래밍/Python
Module import하기 ceil ➡ 올림 fabs ➡ 절대값 fsum ➡ 합계 import math print(math.ceil(1.2)) print(math.fabs(-1.2)) # 2 # 1.2 import math를 하게 되면 사용하지 않는 기능들 모두를 가져오기때문에 필요한 기능만 import하는게 좋음 from math import ceil, fsum print(math.ceil(1.2)) print(math.fsum([1, 2, 3])) # 2 # 6.0 ❓ import할때 fsum의 이름을 변경하고 싶다면 from math import fsum as result_sum print(math.result_sum([1, 2, 3])) # 6.0 내가 정의한 함수를 import하여 사용하고 싶..
조건문 if & 반복문 for
2020. 12. 22. 18:17
프로그래밍/Python
if문 인자 b가 int이거나 float일때만 출력 def plus(a, b): if type(b) is int or type(b) is float: return a + b else: return None print(plus(12, "55")) if문에는 if ~ elif ~ else 사용할 수 있음 for문 python에서는 string도 배열(이론적으로)이기때문에 for문에 사용 가능 days = ("월", "화", "수", "목", "금") for day in days: if day is "수": break else: print(day) ''' 월 화 ''' for num in [1, 2, 3, 4, 5]: print(num) ''' 1 2 3 4 5 ''' for num in "hello": pr..
Function 정의
2020. 12. 21. 13:04
프로그래밍/Python
function 정의하기 python은 들여쓰기로 function의 시작과 끝을 구분하기에 들여쓰기가 아주 중요함! def say_hello(): print("hello") say_hello() # hello 출력 function의 argument(인자) argument에 default value를 줄 수 있음 💡 Positional Argument : 위치에 의존적인 인자(보통 우리가 알고있는 인자) 💡 Keyword Argument : 위치에 따라서 정해지는 인자가 아닌 argument의 이름으로 쌍을 이뤄주는 인자(python의 특징, 자주 사용함💥) def say_hello(who): print("hello", who) say_hello("Eunwoo") # hello Eunwoo 출력 def p..
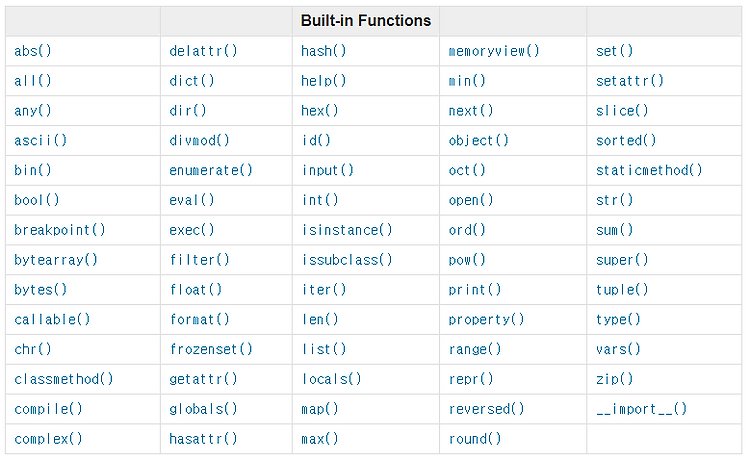
Built in Functions
2020. 12. 21. 12:39
프로그래밍/Python
Built in Functions type을 변경하는 함수 int(), float(), bool(), str() age = "18" print(type(age)) # 출력 n_age = int(age) print(type(n_age)) # 출력 🍋 Built in Functions 참고하기 https://docs.python.org/3/library/functions.html Built-in Functions — Python 3.9.1 documentation Built-in Functions The Python interpreter has a number of functions and types built into it that are always available. They are listed her..
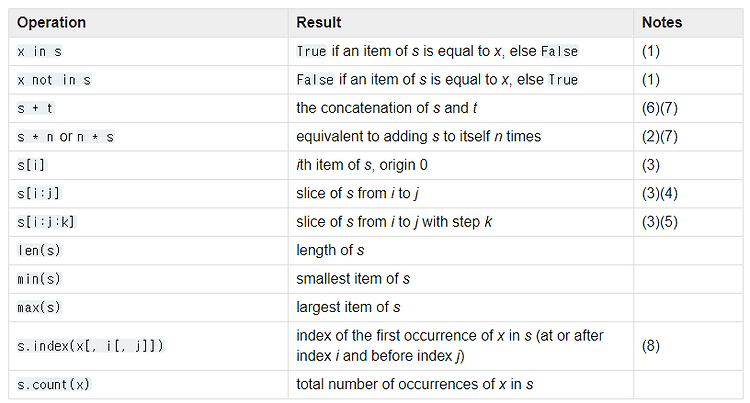
sequence type(열거형 타입) - list, tuple & Dictionary(딕셔너리)
2020. 12. 18. 18:20
프로그래밍/Python
sequence type의 종류 1️⃣ list (Mutable Sequence type) 2️⃣ tuple (Immutable Sequence type) list Common and Mutable Sequence Operations 모두 사용 가능 많은 value들을 열거할 때 사용 📌 3번째 요일을 print하기 days = "Mon,Tue,Wed,Thur,Fri" print(days) 위와 같은 상태로는 3번째 요일을 찾을 수 없음 이때 list가 필요! []로 묶어주고 ,로 value을 나눠주기 days = ["Mon","Tue","Wed","Thur","Fri"] print(days) list 연산자1 value포함 여부, 길이 구하기 days = ["Mon","Tue","Wed","Thur",..
변수
2020. 12. 18. 17:42
프로그래밍/Python
변수 숫자형 변수 a_int = 1 b_float = 2.12 print(a + b) 문자형(String) 변수 ' ' 혹은 " "로 선언해야함 a_string = 'ba' b_string = "nana" print(a + b) 불린형(boolean) 변수 True 혹은 False로 선언하며 따옴표 사용하면 X a_boolean = True b_boolean = False None 변수 파이썬에만 있는 변수로 존재하지 않는다는 뜻(null과 비슷) a_none = None 데이터 타입 확인 방법 a_int = 3 print(type(a_int)) # int 출력 ❓ 변수사용시 _으로 단어를 이어주는 이유(snake case) Python 유저들의 암묵적인 약속으로 변수 이름을 길게 지어야 할때 단어끼리 ..